Do static members ever get garbage collected?
No, static members are associated with the Type, which is associated with the AppDomain it's loaded in.Unless you're considering situations where AppDomains get unloaded, static variables can be regarded as GC roots forever.
Likewise you never set an object to null. You can make the value of a variable null, but that doesn't nothing to any object that the variable previously referred to. It just means that next time the garbage collector looks for live object, that variable won't contribute any object to the set of objects which must be kept alive at the end of the GC.
If you are asking about what happens to objects referenced by variables in static methods, then those objects become elligible for garbage collection when they are no longer in scope.
If you are talking about objects referenced by static fields then these will, in simple terms, not be collected until they the references are set to null.
The following example may illustrate this better:
class Example
{
private static object field1 = new object();
public static void SomeMethod()
{
object variable1 = new object();
// ...
}
public static void Deref()
{
field1 = null;
}
}
The object referenced by field1 will be created when the class is loaded and will remain rooted even as objects of class Example are created and destroyed. The only way to have that object garbage collected would be to call the Deref() method which will dereference it by setting the reference to null. (Actually, it is possible to unload classes by unloading an app domain but this is somewhat more advanced and not something you are likely to come across that often.)
Conversely, the static method SomeMethod() creates an object and references it by the variablevariable1. This object is elligible for garbage collection as soon as it goes out of scope (at the end of the method). Pratically, it could be collected earlier if the remainder of the method does not reference it.
|
Static Variables
1. Variables defined local to a function disappear at the end of the function scope. So when we call the function again, storage for variables is created and values are reinitialized.
2. So if we want the value to be extent throughout the life of a program, we can define the local variable as "static." Initialization is performed only at the first call and data is retained between func calls.
3. Static variables are local in scope to their module in which they are defined, but life is throughout the program. Say for a static variable inside a function cannot be called from outside the function but is alive and exists in memory.
Application Variables
1. Global Variables are also similar to the static variables but these variables are available outside the module/function and its scope is available through out the application.
2. These Variables can be accessed from any of the function
***********************************************************************
When developing Web applications with ASP.NET, you sometimes need to access data which is shared among users again and again throughout the life of the application.
If you want to share a value or an object instance between all sessions, you typically use the Application object. However, a better alternative to Application object is a static property defined in a class. Static properties maintain their values throughout the Application. So they work like the Application object.
Storing and reading a static value is faster when we compare it with the Application object because static variables do not need to look-up in a collection when you refer to them and you do not need to cast from object to a specific type.
The key reason that the Application object exists in ASP.NET is for compatibility with classic ASP code to allow easy migration of existing applications to ASP.NET.
******************************************************************************
memory-leaks-and-dangling-pointers
A1 - Dangling pointer points to a memory location which
is
being removed.
E.g.
int* a = new int;
int *b = a;
delete
b;
Now a will be a dandling pointer.
A2 - Memory leaks occur when
memory allocated to a pointer
is not deleted and the pointer goes out of
scope. This
allocated memory never gets de-allocated and remains in
the
heap until the system is restarted.
E.g.
void foo()
{
int*
ptr = new int;
*ptr = 10;
...
...
}
Since ptr is allocated memory
using 'new', but no 'delete'
is called, hence memory allocated to ptr will
leak.
**************************************************************************
How to identify memory leaks in the common language runtime
Definition of memory leak in C#
Memory leaks can cause an application to run out of resources and can cause an application to crash. It is important to identify memory leaks. The problem of memory leaks has plagued developers in C and C++ for years. In Microsoft Visual Studio 2005 or in Microsoft Visual Studio .NET, a comprehensive garbage collection package and managed memory can stop memory leaks, but, under some circumstances, a program may appear to be leaking memory.Definition of memory leak
A memory leak occurs when memory is allocated in a program and is never returned to the operating system, even though the program does not use the memory any longer. The following are the four basic types of memory leaks:
- In a manually managed memory environment: Memory is dynamically allocated and referenced by a pointer. The pointer is erased before the memory is freed. After the pointer is erased, the memory can no longer be accessed and therefore cannot be freed.
- In a dynamically managed memory environment: Memory is disposed of but never collected, because a reference to the object is still active. Because a reference to the object is still active, the garbage collector never collects that memory. This can occur with a reference that is set by the system or the program.
- In a dynamically managed memory environment: The garbage collector can collect and free the memory but never returns it to the operating system. This occurs when the garbage collector cannot move the objects that are still in use to one portion of the memory and free the rest.
- In any memory environment: Poor memory management can result when many large objects are declared and never permitted to leave scope. As a result, memory is used and never freed.
Discussion
Because of the garbage collection package that is implemented in the Microsoft .NET Framework, it is not possible to have a memory leak in managed code. This suggests two questions: How then can a memory leak occur? Why does it appear that you have a memory leak? A memory leak can occur in a .NET Framework application when you use unmanaged code as part of the application. This unmanaged code can leak memory, and the .NET Framework runtime cannot address that problem. Additionally, a project may only appear to have a memory leak. This condition can occur if many large objects (such asDataTable objects) are declared and then added to a collection (such as a DataSet). The resources that these objects own may never be released, and the resources are left alive for the whole run of the program. This appears to be a leak, but actually it is just a symptom of the way that memory is being allocated in the program. For example, you have a DataSet. Every time that a new query is run, you add a new DataTable element to that DataSet to hold the data that is returned. If there are large amounts of data that you never dispose of, the data stays alive as long as theDataSet is still in use. If this occurs enough times, it is possible to run out of memory. This is not a memory leak, but instead it is a problem in managing the memory. See the following code example:Dim DS As DataSet Dim cn As New SqlClient.SqlConnection("data source=localhost;initial catalog=Northwind;integrated security=SSPI") cn.Open() Dim da As New SqlClient.SqlDataAdapter("Select * from Employees", cn) Dim i As Integer DS = New DataSet() For i = 0 To 1000 da.Fill(DS, "Table" + i.ToString) Next
Note This example is just a snippet of code. This example assumes that Microsoft SQL Server is installed on the local computer and that the user who is running this code has access to the Northwind database that is included with SQL Server. Although this code is obviously inefficient and not practical, it is meant to demonstrate that if objects are added to a collection (such as adding the tables to the DataSet collection), the objects are kept active as long as the collection remains alive. If a collection is declared at the global level of the program, and objects are declared throughout the program and added to that collection, this means that even though the objects are no longer in scope, the objects remain alive because they are still being referenced. Each time that this occurs, the amount of memory that the program is using increases. The memory does not decrease until the end of the program or the release of the objects from the collection. When you watch the program on a performance monitor, this appears to be a memory leak, but it is not. The program still has control over the memory but has chosen not to release it. The fact that the program still has control prevents this from being a memory leak, but the fact that the program keeps increasing the amount of memory used can make it appear to be a memory leak.Symptoms of memory leak
When the amount of memory that a program is using continues to increase during the execution, this is a symptom of a memory leak. (You can watch this count of memory through a performance monitor.) The amount of memory that the program uses can eventually cause the program to run out of resources and to crash.
One of the joys of working with managed code is not having to worry (as much) about memory management and letting the Garbage Collector do it's job. There are situations, however, when applications need to take a more active role in memory management. At MEDC 2005, I spoke, during my debugging session, about three common causes of memory leaks in managed applications:
- Holding references to managed objects
- Failing to release unmanaged resources
- Failing to dispose Drawing objects
Holding references to managed objects I do not actually consider the first of the three causes to be a leak in the truest sense of the term. I say this because the memory in question is accounted for by at least one valid reference within the current scope. While not a "true" leak, this can appear as one when looking at memory usage data. When an application holds references longer than necessary, performance counters can show a steady increase in memory consumption and may, eventually, lead to an OutOfMemoryException. This situation can arise when variables never leave active scope. An example of this is an application that keeps track of large amounts of data in a class global collection. If at least one reference to a managed object remains in the current scope, the Garbage Collector cannot tell if your application is finished with the object. Since, as far as the GC can tell, the object is still in use, it cannot be marked for collection and therefore the memory is not freed. Since the memory is not freed the application's memory consumption continues to grow which looks very much like a memory leak. Whenever possible, I recommend minimizing the scope of an object (local rather than class global variables). You can also let the Garbage Collector know you are finished with an object by setting your variable's value to null (Nothing in Visual Basic.NET) once you are finished using the object. This way, the GC can collect your unused objects at its earliest convenience and your application's memory consumption can be kept to a minimum. Failing to release unmanaged resourcesI mentioned, at the start of this post, that writing managed code largely frees you from needing to be concerned about memory management. This is true when you are using only managed objects. When you interoperate with native APIs (ex: via P/Invoke), your application needs to follow the native code memory management semantics -- loaded objects must be unloaded, allocated memory must be freed, etc. A good example is the original version of the code in my post on getting the display color depth. While the code worked, there was a small memory leak. One of my readers kindly pointed out that I was forgetting to callReleaseDC (I had previously called GetDC). This is a fairly common mistake, and I remember telling myself to not forget the call to ReleaseDC... While forgetting to call ReleaseDC is a relatively small leak, compared to forgetting to unload a large resource, these small leaks can build up quite rapidly. Whenever an application consumes unmanaged resources, I highly recommend a code review with another developer. I have found that walking someone through a block of code forces me to view it from a different angle and allows me to find these mistakes sooner. The best source of information regarding proper handing of unmanaged resources is the API documentation. It has been my experience that the MSDN documentation does a good job at providing cleanup information for resources requiring cleanup, typically in the remarks section of the API reference. Failing to dispose Drawing objects A third common cause of memory leaks in managed applications is actually a manifestation of the previous cause. When consuming System.Drawing and Microsoft.WindowsMobile.DirectX.Direct3D objects, such asbitmaps, fonts, meshes and textures, it is important to call the object's Dispose method when you no longer need the object. This is important because, while these are managed objects, they contain references to unmanaged resources. These references are cleaned up (and the memory used is freed) when the object is disposed. Methods of disposal There are three methods for disposing of objects which require disposal.
- Explicit call to the Dispose method Example:
playerImage->Dispose();
- Implicit via the C# using statement Example:
using(SolidBrush brush = new SolidBrush(Color.Yellow)) { /* use the brush */ }
- Finalization (not recommended)
Of these three disposal methods, two of them warrant further discussion. Implicit disposal (C# using statement) The documentation for the using statement states: "You create an instance in a using statement to ensure that Dispose is called on the object when the using statement is exited." This is a very handy way to ensure that objects requiring disposal get cleaned up properly. One drawback to disposal via the using statement is that all use of the object needs to be contained within the using statement's code block (within the curly braces { }). This is not a very serious drawback, however. In my experience, I rarely have need to use objects containing unmanaged resources (ex: System.Drawing.Bitmap) for more than a few lines of code -- in an OnPaint handler, for example. If your application requires longer lived objects, explicitly calling the Dispose method when finished is the recommended approach. Finalization (not recommended) I do not recommend relying upon finalization to dispose of object requiring disposal for two reasons. The first reason is that the timing of finalization is not guaranteed. Finalization is performed at the discretion of the .NET Compact Framework runtime and can occur at any time in the future. While it is possible that finalization will occur soon after an object requiring disposal has been collected by the GC, it could also occur a significant time later. Secondly, an object's finalizer may not actually invoke its Dispose method. I would consider an object not calling Dispose as part of finalizationto contain a bug. While the bug would be in the object, it is the application which will likely get the report of the memory leak. For a good discussion on implementing Dispose for your objects using unmanaged resources, please readImplementing Finalize and Dispose to Clean Up Unmanaged Resources (on MSDN). This post has covered three common causes of memory leaks in managed applications. If you believe your application is encountering a leak, and you have been able to rule out these causes, please let us know about the issue via the Microsoft Product Feedback Center.What Is Managed Unmanaged in NET Framework.
The term "unmanaged resource" is usually used to describe something not directly under the control of the garbage collector. For example, if you open a connection to a database server this will use resources on the server (for maintaining the connection) and possibly other non-.net resources on the client machine, if the provider isn't written entirely in managed code.This is why, for something like a database connection, it's recommended you write your code thusly:using (var connection = new SqlConnection("connection_string_here")) { // Code to use connection here }
As this ensures that.Dispose()
is called on the connection object, ensuring that any unmanaged resources are cleaned up.The basic difference between a managed and unmanaged resource is that the garbage collector knows about all managed resources, at some point in time the GC will come along and clean up all the memory and resources associated with a managed object. The GC does not know about unmanaged resources, such as files, stream and handles, so if you do not clean them up explicitly in your code then you will end up with memory leaks and locked resources. The idea behind the IDisposable interface is to let you clean up resources in a deterministic fashion and clean up unmanaged resources. Even if you do not have unmanaged resources in your class, you may have large managed resources like images (which you mentioned) which are taking up valauable resources such as memory, network connections etc, if you no longer need these objects then it is nice to clean up all of the resources associated with the object as soon as possible, rather than waiting until some indeterminate point in the future when the GC gets called. This code is placed inside the Dispose functions. In answer to one of your questions, if you see that an object implements the IDisposable interface i.e. it has a "public void Dispose()" method then this is an indication that either the object has unmanaged resources that need cleaning up or it has some other resources that should be clean up sooner than later (i.e. by waiting for the garbage collector) and you should call the Dispose() method when you are finished with the object. Also anytime you implement the Dispose function you also want to make sure that you call the Dispose function from your objects finalizer. This is because you cannot force someone to call your Dispose function, so if they do not call it then you need to make sure the GC calls it instead. On the other flip side, if the user calls Dispose() then you can stop the GC from calling the dispose function in the finalizer because this is unneccessary and having a finalizer on an object is an expensive operation. You can do this by calling GC.SupressFinalize(this), which makes sure the object does not get put on the GC's finalize queue. In answer to another one of your questions, the Image class has unmanaged references inside it, but the Image class itself is a managed wrapper around these items. It has a public Dispose function and a Finalizer which calls Dispose incase you forget to call it. Therefore even if you don't call Dispose then unmanaged resources will eventually get cleared up when the GC calls the objects finalizer method. The big point to note here is WHEN the GC calls the finalizer. You really have no way to tell when this is going to happen, so the reason you want to call dispose on the Image object is because you are using up a lot of memory resources, if you no longer need the object then calling dipose will free these resources immediately, rather than waiting for the GC to run.public class ImgViewer : UserControl { // bmp can get a value in response to some user actions private Bitmap bmp; protected override void Dispose( bool disposing ) { if( disposing ) { if (components != null) { components.Dispose(); } } if ( bmp != null ) bmp.Dispose(); base.Dispose( disposing ); } } Although not technically wrong because you are checking the object against null, calls to methods on managed objects should be inside the if(disposing) statement. The basic Dispose pattern used goes something like this: class MyObject : IDisposable { //indicates if dispose has already been called //private bool _disposed = false; //Finalize method for the object, will call Dispose for us //to clean up the resources if the user has not called it ~MyObject() { //Indicate that the GC called Dispose, not the user Dispose(false); } //This is the public method, it will HOPEFULLY but //not always be called by users of the class public void Dispose() { //indicate this was NOT called by the Garbage collector Dispose(true); //Now we have disposed of all our resources, the GC does not //need to do anything, stop the finalizer being called GC.SupressFinalize(this); } private void Dispose(bool disposing) { //Check to see if we have already disposed the object //this is necessary because we should be able to call //Dispose multiple times without throwing an error if(!disposed) { if(disposing) { //clean up managed resources } //clear up any unmanaged resources - this is safe to //put outside the disposing check because if the user //called dispose we want to also clean up unmanaged //resources, if the GC called Dispose then we only //want to clean up managed resources } } } The boolean disposing indicates whether or not the private dispose method was called by the user or by the Garbage collector. There is an important reason for this. If the user calls Dispose then you know that all managed resources pointed to by your object must still be alive i.e. not garbage collected because your object still has a reference to them so they will not be collected and someone must have had a reference to your object to call the public Dispose() method. If the GC called Dispose then you cannot be sure that the objects which your object is referencing have not already been garbage collected, so you should not call any methods on them. You can be sure that unmanaged resources have not been Garbage collected because the GC does not know about them so it is safe to clean them up. If you are inheriting then you need to make the Dispose(bool disposing) method virtual and make sure you call it from the base class to make sure resources in the base class are disposed correctly.Managed resources basically means "managed memory" that is managed by the garbage collector. When you no longer have any references to a managed object (which uses managed memory), the garbage collector will (eventually) release that memory for you.Unmanaged resources are then everything that the garbage collector does not know about. For example:
- Open files
- Open network connections
- Unmanaged memory
- In XNA: vertex buffers, index buffers, textures, etc.
Normally you want to release those unmanaged resources before you lose all the references you have to the object managing them. You do this by calling
Dispose
on that object, or (in C#) using the using
statement which will handle calling Dispose
for you.
If you neglect to
Dispose
of your unmanaged resources correctly, the garbage collector will eventually handle it for you when the object containing that resource is garbage collected (this is "finalization"). But because the garbage collector doesn't know about the unmanaged resources, it can't tell how badly it needs to release them - so it's possible for your program to perform poorly or run out of resources entirely.
If you implement a class yourself that handles unmanaged resources, it is up to you to implement
Dispose
and Finalize
correctly.
If you are not calling Dispose method , Than CLR will default call Finalize method which will reclaim the memory But point is we dont know when CLR will(Destructer ==Finalize) Because GC runs when it memory is really needed by the program, or you can say when perforamnce will start decresing than only it will start .So better that when you know your object using any unmanaged resourse make sure call Dispose or Implement IDisposable interface in your class. In c, C++ we dont have this feature so it is programmer responsibility that he is releasing memory so that no Memory leak or Dangling pointer happens. IN NET we have GC feature so we never bother about the reclaming memory but if you know that your program use Unmanged API or OLD COM API than take care for that since GC will taking care of any Unmanaged Code.
******************************************************************************************
****************************************************************************************
Managed code and unmanaged code in .NET
Managed resources basically means "managed memory" that is
managed by the garbage collector. When you no longer have any references to a
managed object (which uses managed memory), the garbage collector will
(eventually) release that memory for you.
Unmanaged resources are then everything that the garbage collector does
not know about. For example:
·
Open files
·
Open network connections
·
Unmanaged memory
·
In XNA: vertex buffers, index buffers, textures, etc.
Normally you want to release those
unmanaged resources before you lose
all the references you have to the object managing them. You do this by
calling Dispose on that object, or (in C#) using the usingstatement which will
handle calling Dispose for you.
If you neglect to Dispose of your
unmanaged resources correctly, the garbage collector will eventually handle it
for you when the object containing that resource is garbage collected (this is
"finalization"). But because the garbage collector doesn't know about
the unmanaged resources, it can't tell how badly it needs to release them - so
it's possible for your program to perform poorly or run out of resources
entirely.
If you implement a class yourself that
handles unmanaged resources, it is up to you to implementDispose and Finalize correctly.
Open
Database connection comes under which category? Managed / Unmanaged?
the important point that you are calling Dispose on a managed object that internally handles the
freeing up of the unmanaged resource it wraps (e.g. file handle, GDI+ bitmap,
...) and that if you access unmanaged resources directly (PInvoke etc.) you
need to handle that.
as the GC
doesn't know about it (assuming you are not using some hypothetical
in-managed-memory database). But the connection object itself might not hold an unmanaged
resource. Presumably the database connection uses an open file or network
connection somewhere - but it is possible that another object (other
than the connection object) is handling that unmanaged resource (perhaps your
database library caches connections). Check the documentation and see where it
asks you to call
Dispose
or use using
. –
I have a basic
comment/question on this, can I relate an object as managed/un-managed just by
the type, for example, string is managed, DataSet is un-managed (which is why
it has a Dispose() method), Database connections are un-managed (because they
have dispose), etc. So is the assumption, if it has a "Dispose()"
method, then it's un-managed? In addition to that, what would an XmlDocument
object be.
Although be mindful that
all C# class instances are managed objects. If an instance of a class could hold unmanaged resources, then that
class should implement
IDisposable
. If a class does implement IDisposable
, then you should dispose
of instances of that class with using
or Dispose()
when you are done with them. Based on this,
your converse holds: If a class implements IDisposable
, then it probably holds
unmanaged resources internally.
Unmanaged resources are
those that run outside the .NET runtime (CLR)(aka non-.NET code.) For example,
a call to a DLL in the Win32 API, or a call to a .dll written in C++..
The .Net Framework provides a new mechanism for
releasing unreferenced objects from the memory (that is we no longer needed
that objects in the program) ,this process is called Garbage Collection (GC).
When a program creates an Object, the Object takes up the memory. Later when
the program has no more references to that Object, the Object's memory becomes
unreachable, but it is not immediately freed. The Garbage Collection checks to
see if there are any Objects in the heap that are no longer being used by the
application. If such Objects exist, then the memory used by these Objects can
be reclaimed. So these unreferenced Objects should be removed from memory ,
then the other new Objects you create can find a place in the Heap.
The reclaimed Objects have to be
Finalized later. Finalization allows a resource to clean up after itself when
it is being collected. This releasing of unreferenced Objects is happening
automatically in .Net languages by the Garbage Collector (GC). The programming
languages like C++, programmers are responsible for allocating memory for Objects
they created in the application and reclaiming the memory when that Object is
no longer needed for the program. In .Net languages there is a facility that we
can call Garbage Collector (GC) explicitly in the program by calling
System.GC.Collect.
Be Careful with Virtual Method Calls from the
Constructor (and Destructor) of your Classes in C++/C#/Java!
The Order
of Constructor/Destructor Calls in a Class Hierarchy
For demonstration, I will use the following class hierarchies:

Constructor call order is the following in case of constructing
a
Derived2
instance (for all 3 languages):
1.
Base
2.
Derived1
3.
Derived2
Destructor call order in case of C++ is the reverse of
constructor call order:
1.
~Derived2
2.
~Derived1
3.
~Base
The
construction of an instance always starts with allocating/reserving a memory
block for it before calling the constructor of the class being instantiated.
In below example I created the object of derived class and
checked that above statement is true. Derived class memory have been allocated
and variable also get initialize before initializing base member variable they
are initialize with default values, however y1 object does not exist. Y1 exist
after calling and Base class constructer and control return to the last
position.
In main when compiler
executes y y1=new y() , than it will initialize the class member of Y with 8
and than it goes to class X initilzes the X clss variable.
using System;
using System.Collections.Generic;
using System.Text;
namespace IDisposableTestApplication
{
class X
{
private int m = 0;
private int n = 90;
public X() { }
}
class Y:X
{
private int i = 8;
private int j = 6;
public Y()
{ }
public static void Main(string[]
args)
{
Y y1 = new Y();
}
}
}



constructor()
{
auto generated: initialize the member
variables (fields) of this class
auto generated: call the base
class
constructor (if we have a base
class)
[your constructor code here]
}
namespace IDisposableTestApplication
{/*Destructer calles at
last in the program.IF we remove console.Readline() from destructer thaen I can
not see output dont know wen it get called in the program.
* Destructor is invoked whenever an object is about to be garbage
collected.
*/
class A
{
public A() { Console.WriteLine("BASEA constructer"); }
~A()
{ Console.WriteLine("BASEA
Destructer"); Console.ReadLine();
} //Error 1 The modifier 'public' is not valid for
this item
}
class B : A
{
public B() { Console.WriteLine("Child B constructer"); }
~B()
{ Console.WriteLine("Child
B Destructer"); Console.ReadLine();
}
}
class C
{
public static void Main(string[]
args)
{
B b = new B();
Console.ReadLine();
}
}
}

Difference between Destructor and Finalize()
method in C#
Difference between Destructor and Finalize()
method in C#
Finalize() Method of Object class
Each class in C# is automatically
(implicitly) inherited from the Object class which contains a method
Finalize(). This method is guaranteed to be called when your object is garbage
collected (removed from memory). You can override this method and put here code
for freeing resources that you reserved when using the object.
Example of Finalize Method
Protected override void Finalize()
{
try
{
Console.WriteLine(“Destructing
Object….”);//put some code here.
}
finally
{
base.Finalize();
}
}
Destructor
1) A destructor is just opposite to constructor.
2) It has same as the class name, but with prefix ~ (tilde).
3) They do not have return types, not even void and therefore they cannot
return values.
4) Destructor is invoked whenever an object is about to be garbage collected.
Example of Destructor in .Net
class person
{
//constructor
person()
{
}
//destructor
~person()
{
//put resource freeing code here.
}
}
Difference between the destructor and the Finalize()
method
Finalize() corresponds to the .Net
Framework and is part of the System.Object class. Destructors are C#'s
implementation of the Finalize() method.
The functionality of both Finalize()
and the destructor is the same, i.e., they contain code for freeing the
resources when the object is about to be garbage collected.
In C#, destructors are converted to
the Finalize() method when the program is compiled.
The Finalize() method is called by
the .Net Runtime and we cannot predict when it will be called. It is guaranteed
to be called when there is no reference pointing to the object and the object
is about to be garbage collected.
namespace IDisposableTestApplication
{
class A1
{
public A1() { Console.WriteLine("BASEA constructer"); }
~A1()
{ Console.WriteLine("BASEA
Destructer"); Console.ReadLine();
Console.ReadLine(); Console.ReadLine();
} //Error 1 The modifier 'public' is not valid for
this item
}
class B1 : A1
{
public B1() { Console.WriteLine("Child B constructer"); }
// ~B1() {
Console.WriteLine("Child B Destructer"); Console.ReadLine(); }
protected virtual void Finalize() //If both
Finalize and des in same class that getting errorType
'IDisposableTestApplication.B1' already defines a member called 'Finalize' with
the same parameter types, So I removed des from B and implement finalize. Now
since in I dont have Finalize in A1 so i called des in A1
{
try
{
Console.ReadLine(); Console.ReadLine(); Console.ReadLine();
Console.WriteLine("Destructing Object….");//put some code here.
Console.ReadLine(); Console.ReadLine(); Console.ReadLine();
}
finally
{
//
base.Finalize();//Do not directly call your base class Finalize method.
It is called automatically from your destructor. Since pattern of des are opp
to cons. this.Finalize();
}
}
}
class C1
{
public static void Main(string[]
args)
{
B1 b = new B1();
Console.ReadLine();
}
}
}
We know the sequence of des, and it child obj and than base here
in output we see the b


In
above program we have write Finalize that is not the same of GC fianlize or
Object class fianize, .net framework not allows us to do that. But if you write
base.Fianlize //Do not directly call your base class Finalize method. It is
called automatically from your destructor, it says like that means In the end
of program Fianlize called.
So we can have destructor in our class
instead of Finalize and if we see the IL code it turns into try
finally{Finalize}, and GC.
*********************************************************************************************
*****************************************************************************************
CLR’s style is referred as “non-deterministic memory management
Why do you a need a Finalizer?
IDisposable for Dummies You only need to implement a Finalizer (i.e. override Finalize() method) in your class ifyour class directly creates a native resource (aka unmanaged resource) like a memory space in the unmanaged heap, a GDI handle or a file handle.
Otherwise, if your class is using the .NET objects provided by the .NET framework (e.g.System.IO. Stream), those classes are managed resources and they already implement IDisposable and have a Finalize() method overridden. So, you only need to call their Dispose() method from your implementation. YOU DO NOT HAVE TO implement a Finalizer() in that case.
So that means Ultimetly Finalize will run to relcaime the memory.
IDisposable for Dummies #1 – Why? What?
It took me more than a year to finish writing this article – a “hot topic” and I wanted it to be clear, simple and right. Also, I was busy and did not want to publish anything “unfinished”. As usual, your feedback and comments are more than welcome. Thank you in advance)
Recently (February 2011), I had to review some .NET code and came across of some wrong implementations of IDisposable.
After discussing with the developers, there were many reasons for what I found, ranging from:
- Not knowing the difference between a “CLR memory resource”, a “managed resource” and an “unmanaged resource”;
- Not understanding “how and when” resources are “released”;
- Not knowing when to override the “Finalize()” method and what should be released by a finalizer?
Even if the recent publications are becoming better, documentation from Microsoft is not fully clear on this subject. There are still some ambiguous areas about when you should implement a Finalizer (I must admit, since I started to do .NET programming back in 2002 (with .NET 1.1), MSDN documentation has improved a lot, but it took me a lot of time to understand this topic – thanks to the books like the ones from Jeffrey Richter [REF-01], Don Box [REF-02] and Bill Wagner [REF-03]).
I have split this matter into two posts :
- # 1 – What + Why + references (this post)
- #2 – A guide about ‘how to implement it’ + samples
If you already know about the IDisposable pattern and what are the types of resources managed by the .NET CLR (Common Language Runtime – Microsoft implementation of the managed runtime environment on the .NET platform), please go directly to the second post. If you do not know or want to learn more about it, please keep reading.
What are memory resources on .NET CLR?
In managed environments (like the .NET CLR or the Java JVM), “memory management on the heap” is taken care by the runtime engine, i.e. CLR on the .NET platform – The rest of the discussion will focus only on the .NET platform.
Like a supervisor, the runtime engine knows everything about heap memory allocation in its world, aka the managed environment. However, this managed environment lives and is hosted in a less “managed” environment, e.g. the Windows operating system. Your application might need some resources that are not managed by the CLR and even living outside your process. They could be locally allocated on the same machine or remotely far away on another machine. The important point is that you can use them from your code in .NET.
From this picture we can see 3 types of memory resources for a .NET application:
- CLR memory resources, such as _myNetObj or the string “Hello world!”. Their memory space and fields (int and string) are all allocated on the CLR’s heap memory.
- Managed resources, such as _myObjWindow, _myObjFile, _timer1, _file1,_connection1. They are .NET classes which have direct unmanaged fields and/orother managed resource as fields. Their memory space is managed by the CLR.
- Unmanaged resources, such as window, timer, file, DB. They live outside the CLR’s heap memory. They can be referred as “native resources” as well.
The CLR only controls and manages its memory. The CLR has its own algorithms to allocate/move/release its memory (heap, large object heap, …). The CLR’s heap memory management is based on a “generational garbage collection”. I am not digging too much in details inside the CLR implementation of its “garbage collector”, because it would require many posts
, but the aim of the garbage collector is to reclaim not reachable managed memory and re-compact the memory to prevent memory fragmentation as much as possible. For further details, please see [REF-01] [REF-07] and [REF-08].
The bold statement above requires a shift of mind from the way development is done in a “native memory management”, such as in C and C++ language, compare to a managed environment like the .NET CLR.
In native memory management, the developer that you are is in charge of the memory allocation, you will do a malloc() (or new()) method to allocate memory, use your object and when you do not need it anymore you will call de-allocate() (or delete()) method to free the memory. You have “great powers”, but also great responsibilities about the management of your memory. This style of memory management is also referred as “deterministic memory management“. By opposition, CLR’s style is referred as “non-deterministic memory management“.
In the “deterministic memory management” style, there are a few drawbacks:
- First you end up managing handlers/pointers to memory area. You have to be careful and aware of the size of the “right portion of the memory allocated” when writing and reading from it.
- Overrun buffer, stack data corrupted, memory corrupted are results of misuse of those handlers/pointers.
- For some applications, the repetition of the cycle “allocation/de-allocation” on a native heap space will fragment the memory and the process will end up at some point with an “out-of-memory” error, even though it still has a lot of free space (the free space is fragmented and not contiguous).
- From a security point of view, the system cannot verify the stack and its memory pointers and apply security checks/rules.
To address some of those issues, managed environments (which have existed since the 70s, “hello Smalltalk !”) have been revisited and refreshed with the latest features. For the CLR, this includes the following features (not a thorough list): “generational garbage collectors” and “Code Access Security (CAS) for .NET”.
Now, in this new managed environment world, the developers are not concerns anymore about memory allocation/de-allocation of CLR memory resources. However, as your application does not lived (yet!) in a full managed operating system, you must pay attention to managed resources and unmanaged resources.
The other key point to retain is that once you have finished using an object allocated on the CLR’s heap it might be de-allocated at some point but nothing is certain and there is no guaranty (i.e. about “when” and whether it would be de-allocated at all).
In fact, in the “non-deterministic memory management” style you have:
- Not sure the CLR will re-use the memory space used be your object (if there is no need to claim memory, why bother).
- You do not know when this space will be re-used.
That leaves the .NET developer with 2 problems:
- How can he “force/trigger” the release of resources?
- How can he make sure that managed resources and unmanaged resources will still be released (at some point)?
To help you with that task about releasing other resources than CLR memory resources, the .NET team came up with the IDisposable interface [Ref-04] and the Dispose pattern [Ref-05]. The other .NET feature that will help you is the Finalize() method (a protected method define at the System.Object level).
What about IDisposable ?
.NET objects live in a managed world (aka CLR). We know that the CLR memory management style is non-deterministic, i.e. developers do not have to worry about releasing the CLR heap memory allocated by CLR memory resources. However, for other types, managed resources and unmanaged resources, we do not want those objects to stay in memory for long after we have finished to use them. Some of those objects have a Close() method, some have a Free() method, some have a Release() method, and some have even a mix of them.
So, how can I (as a developer) know which one to call to free up the managed and native resources? (like DB connections, files, …)
The .NET team came up with a single interface named IDisposable with a single method called Dispose() to standardize the way resources are disposed:
- Because it is an interface, it does not prevent your class to inherit implementation from whatever class it needs to (remember, you are only allow one implementation class inheritance, but many interface inheritances);
- As you decorate your class with that interface, it adds semantics (interfaces are like contracts) and intention to your class. So, developers using your class should know about it.
- The dispose method is simple – no parameters – and its name is clear: dispose any resources you have.
- Developers should only be aware if a class Implements IDisposable to call a single method, Dispose(), to release all resources by an instance of that class.
The .NET C# team went a step further by providing a construct in the language called “using” that will allow you to declare, use and call the Dispose() method in atry/finally block.
Why do you a need a Finalizer?
You only need to implement a Finalizer (i.e. override Finalize() method) in your class ifyour class directly creates a native resource (aka unmanaged resource) like a memory space in the unmanaged heap, a GDI handle or a file handle.
Otherwise, if your class is using the .NET objects provided by the .NET framework (e.g.System.IO. Stream), those classes are managed resources and they already implement IDisposable and have a Finalize() method overridden. So, you only need to call their Dispose() method from your implementation. YOU DO NOT HAVE TO implement a Finalizer() in that case.
Jeffrey Ritcher [Ref-01] has some other examples that you might want to implement a Finalizer() in your class even though your class does not have a (direct) native resource (e.g. if you want to monitor garbage collection). I do think this is the exception to the rule.
In the “non-deterministic memory management” style the finalization is as follows:
- Exact time of finalization is unspecified. (When? => you do not know)
- Order of finalization is unspecified. (Order? => you do not know)
- Thread running the Finalize() method is unspecified. (Which Thread? => you do not know)
Objects with a Finalize() method overridden have an extra cost associated with them in order to reclaim the CLR memory used by your object [REF-01][REF-06][REF-07][REF-08] . It will take at least two garbage collections:
- First one to put your object in the “f-reachable” list;
- Run your Finalizer() code
- Then remove your object from the “f-reachable” list, so that your object becomes unreachable (i.e. no roots referencing it);
- Its managed heap memory will be reclaimed in the next full garbage collection(i.e. generation 2, current maximum generation level in the CLR as I am writing these lines).
The .NET C# team provided a destructor syntax (only the syntax, not the semantics !!), but the piece of code written in that method will be placed inside the Finalize()method by the C# compiler in a try/finally block, with a call to the base class in the finally block. This is maybe one of the ambiguous area and syntax for C# developers to understand: the Finalize() method is not a destructor, i.e. like in C++.
If your class has one (or more) unmanaged resource then you will need to:
- Override the Finalize() method;
- Call GC.SuppressFinalize() from your public Dispose() method;
- Your Dispose() method must release any managed and unmanaged resources;
- Your Dispose() method can be called multiple times without failing.
- Once your object has been disposed, it cannot be (re-)used anymore. It is garbage !!
- Once your object has been disposed, all public instance methods should raise the exception “ObjectDisposedException”.
- Only release unmanaged resources in your Finalize() method.
Please, refer to the second post, there is a detailed matrix about what needs to be implemented in various scenarios and some sample code.
End of part 1
I hope that by now you have a better understanding about:
- The different types of memory resources available in the CLR (CLR memory resource, managed resource and unmanaged resource).
- The IDisposable interface.
- The Finalize() method and when to implement it.
My second post has a detailed matrix to help you find out, depending in your context, what needs to be implemented and some code samples.
References:
- [REF-01] Jeffrey Richter, CLR via C#, 3rd Edition, Microsoft Press (see Chapter 21).
- [REF-02] Don Box and Chris Sells, Essential .NET – Volume 1 (p. 147-152).
- [REF-03] “Effective C#”, Bill Wagner, second edition, Addison Wesley (Ch2 p.69-74; item-17)
- [REF-04] “IDisposable Interface“, MSDN online
- [REF-05] “Implementing a Dispose Method“, MSDN online
- [REF-06] “Finalize Methods and Destructors“, MSDN online
- [REF-07] “Garbage Collection: Automatic Memory Management in the Microsoft .NET Framework (Part-1)“, Jeffrey Richter, MSDN November 2000.
- [REF-08] “Garbage Collection: Automatic Memory Management in the Microsoft .NET Framework (Part-2)“, Jeffrey Richter, MSDN December 2000.
********************************************************************************************
Defines a method to release allocated resources
The primary use of this interface is to release unmanaged resources. The garbage collector automatically releases the memory allocated to a managed object when that object is no longer used. However, it is not possible to predict when garbage collection will occur. Furthermore, the garbage collector has no knowledge of unmanaged resources such as window handles, or open files and streams.
Finalize Methods and Destructors
For the majority of the objects that your application creates, you can rely on the .NET Framework's garbage collector to implicitly perform all the necessary memory management tasks. However, when you create objects that encapsulate unmanaged resources, you must explicitly release the unmanaged resources when you are finished using them in your application. The most common type of unmanaged resource is an object that wraps an operating system resource, such as a file, window, or network connection. Although the garbage collector is able to track the lifetime of an object that encapsulates an unmanaged resource, it does not have specific knowledge about how to clean up the resource. For these types of objects, the .NET Framework provides the Object.Finalize method, which allows an object to clean up its unmanaged resources properly when the garbage collector reclaims the memory used by the object. By default, theFinalize method does nothing. If you want the garbage collector to perform cleanup operations on your object before it reclaims the object's memory, you must override the Finalize method in your class. You can implement the Finalize method when developing in programming languages other than C# and the Managed Extensions for C++. C# and the Managed Extensions provide destructors as the simplified mechanism for writing finalization code. Destructors automatically generate a Finalize method and a call to the base class's Finalize method. You must use destructor syntax for your finalization code in the C# and the Managed Extensions programming languages.
The garbage collector keeps track of objects that have Finalize methods, using an internal structure called the finalization queue. Each time your application creates an object that has a Finalize method, the garbage collector places an entry in the finalization queue that points to that object. The finalization queue contains entries for all the objects in the managed heap that need to have their finalization code called before the garbage collector can reclaim their memory.
Implementing Finalize methods or destructors can have a negative impact on performance and you should avoid using them unnecessarily. Reclaiming the memory used by objects with Finalize methods requires at least two garbage collections. When the garbage collector performs a collection, it reclaims the memory for inaccessible objects without finalizers. At this time, it cannot collect the inaccessible objects that do have finalizers. Instead, it removes the entries for these objects from the finalization queue and places them in a list of objects marked as ready for finalization. Entries in this list point to the objects in the managed heap that are ready to have their finalization code called. The garbage collector calls the Finalize methods for the objects in this list and then removes the entries from the list. A future garbage collection will determine that the finalized objects are truly garbage because they are no longer pointed to by entries in the list of objects marked as ready for finalization. In this future garbage collection, the objects' memory is actually reclaimed.
Implementing a Dispose Method
http://msdn.microsoft.com/en-us/library/fs2xkftw%28v=VS.90%29.aspx
The pattern for disposing an object, referred to as a dispose pattern, imposes order on the lifetime of an object.
A type's Disposemethod should release all the resources that it owns. It should also release all resources owned by its base types by calling its parent type's Dispose method. The parent type's Dispose method should release all resources that it owns and in turn call its parent type's Dispose method, propagating this pattern through the hierarchy of base types. To help ensure that resources are always cleaned up appropriately, a Dispose method should be callable multiple times without throwing an exception.
There is no performance benefit in implementing the Dispose method on types that use only managed resources (such as arrays) because they are automatically reclaimed by the garbage collector. Use the Dispose method primarily on managed objects that use native resources and on COM objects that are exposed to the .NET Framework. Managed objects that use native resources (such as the FileStream class) implement the IDisposable interface.
Important noteImportant Note:
C++ programmers should not use this topic. Instead, see Destructors and Finalizers in Visual C++. In the .NET Framework version 2.0, the C++ compiler provides support for implementing deterministic disposal of resources and does not allow direct implementation of the Dispose method.
A Dispose method should call the SuppressFinalize method for the object it is disposing. If the object is currently on the finalization queue, SuppressFinalize prevents its Finalize method from being called. Remember that executing a Finalize method is costly to performance. If your Dispose method has already done the work to clean up the object, then it is not necessary for the garbage collector to call the object's Finalize method.
The code example provided for the GC.KeepAlive method shows how aggressive garbage collection can cause a finalizer to run while a member of the reclaimed object is still executing. It is a good idea to call the KeepAlive method at the end of a lengthy Dispose method.
Example
The following code example shows the recommended design pattern for implementing a Dispose method for classes that encapsulate unmanaged resources.
Resource classes are typically derived from complex native classes or APIs and must be customized accordingly. Use this code pattern as a starting point for creating a resource class and provide the necessary customization based on the resources you are encapsulating.
C#VB
using System;
using System.IO;
class Program
{
static void Main()
{
try
{
// Initialize a Stream resource to pass
// to the DisposableResource class.
Console.Write("Enter filename and its path: ");
string fileSpec = Console.ReadLine();
FileStream fs = File.OpenRead(fileSpec);
DisposableResource TestObj = new DisposableResource(fs);
// Use the resource.
TestObj.DoSomethingWithResource();
// Dispose the resource.
TestObj.Dispose();
}
catch (FileNotFoundException e)
{
Console.WriteLine(e.Message);
}
}
}
// This class shows how to use a disposable resource.
// The resource is first initialized and passed to
// the constructor, but it could also be
// initialized in the constructor.
// The lifetime of the resource does not
// exceed the lifetime of this instance.
// This type does not need a finalizer because it does not
// directly create a native resource like a file handle
// or memory in the unmanaged heap.
public class DisposableResource : IDisposable
{
private Stream _resource;
private bool _disposed;
// The stream passed to the constructor
// must be readable and not null.
public DisposableResource(Stream stream)
{
if (stream == null)
throw new ArgumentNullException("Stream in null.");
if (!stream.CanRead)
throw new ArgumentException("Stream must be readable.");
_resource = stream;
_disposed = false;
}
// Demonstrates using the resource.
// It must not be already disposed.
public void DoSomethingWithResource() {
if (_disposed)
throw new ObjectDisposedException("Resource was disposed.");
// Show the number of bytes.
int numBytes = (int) _resource.Length;
Console.WriteLine("Number of bytes: {0}", numBytes.ToString());
}
public void Dispose()
{
Dispose(true);
// Use SupressFinalize in case a subclass
// of this type implements a finalizer.
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
// If you need thread safety, use a lock around these
// operations, as well as in your methods that use the resource.
if (!_disposed)
{
if (disposing) {
if (_resource != null)
_resource.Dispose();
Console.WriteLine("Object disposed.");
}
// Indicate that the instance has been disposed.
_resource = null;
_disposed = true;
}
}
}
************************************************************************************
Object.Finalize Method
Allows an Object to attempt to free resources and perform other cleanup operations before the Object is reclaimed by garbage collection.
[C#] In C#, finalizers are expressed using destructor syntax.
Finalize is protected and, therefore, is accessible only through this class or a derived class.
This method is automatically called after an object becomes inaccessible, unless the object has been exempted from finalization by a call to SuppressFinalize. During shutdown of an application domain, Finalize is automatically called on objects that are not exempt from finalization, even those that are still accessible. Finalize is automatically called only once on a given instance, unless the object is re-registered using a mechanism such as ReRegisterForFinalize and GC.SuppressFinalize has not been subsequently called.
Every implementation of Finalize in a derived type must call its base type's implementation of Finalize. This is the only case in which application code is allowed to call Finalize.
Finalize operations have the following limitations:
The exact time when the finalizer executes during garbage collection is undefined. Resources are not guaranteed to be released at any specific time, unless calling a Close method or a Dispose method.
The finalizers of two objects are not guaranteed to run in any specific order, even if one object refers to the other. That is, if Object A has a reference to Object B and both have finalizers, Object B might have already finalized when the finalizer of Object A starts.
The thread on which the finalizer is run is unspecified.
The Finalize method might not run to completion or might not run at all in the following exceptional circumstances:
Another finalizer blocks indefinitely (goes into an infinite loop, tries to obtain a lock it can never obtain and so on). Because the runtime attempts to run finalizers to completion, other finalizers might not be called if a finalizer blocks indefinitely.
The process terminates without giving the runtime a chance to clean up. In this case, the runtime's first notification of process termination is a DLL_PROCESS_DETACH notification.
The runtime continues to Finalize objects during shutdown only while the number of finalizable objects continues to decrease.
If Finalize or an override of Finalize throws an exception, the runtime ignores the exception, terminates that Finalize method, and continues the finalization process.
Notes to Implementers:
Object.Finalize does nothing by default. It must be overridden by a derived class only if necessary, because reclamation during garbage collection tends to take much longer if a Finalize operation must be run.
If an Object holds references to any resources, Finalize must be overridden by a derived class in order to free these resources before the Object is discarded during garbage collection.
A type must implement Finalize when it uses unmanaged resources such as file handles or database connections that must be released when the managed object that uses them is reclaimed. See the IDisposable interface for a complementary and more controllable means of disposing resources.
Finalize can take any action, including resurrecting an object (that is, making the object accessible again) after it has been cleaned up during garbage collection. However, the object can only be resurrected once; Finalize cannot be called on resurrected objects during garbage collection.
[C#] Destructors are the C# mechanism for performing cleanup operations. Destructors provide appropriate safeguards, such as automatically calling the base type's destructor. In C# code, Object.Finalize cannot be called or overridden.
Requirements
Platforms: Windows 98, Windows NT 4.0, Windows Millennium Edition, Windows 2000, Windows XP Home Edition, Windows XP Professional, Windows Server 2003 family, .NET Compact Framework, Common Language Infrastructure (CLI) Standard
IDisposable Interface
Classes that Implement IDisposable
SqlCeCommand Represents an SQL statement to execute against a data source.
SqlCeConnection Represents an open connection to a data source.
SqlCeDataReader Provides a way of reading a forward-only stream of data rows from a data source. This class cannot be inherited.
***************************************************************************************
Introduction
In this article, we will discuss the often confused topic of Implementing the
IDisposable
interface. We will try to see when should be need to implement the IDisposable
interface and what is the right way of implementing it.Background
Often in our application development we need to aquire some resources. These resources coould be files that we want to use from a clustered storage drive, database connections etc. The important thing to remember while using these resources is to release these resources once we are done with it.
C++ programmers are very well aware of the the concept of
Resource Acquisition is Initialization(RAII)
. This programing idiom states that of an object want to use some resources then it is his responsibility to acquire the resource and release it. It should do so in normal conditions and in exceptional/error conditions too. This idiom is implemented very beautifully using constructors, destructors and exception handling mechanism.
Let us not digress and see how we can have a similar idiom in C# using
IDisposable
pattern.Using the code
Before looking into the details of
IDisposable
interface let us see how we typically use the resources, or what are the best practices to use the resources. We should always acquire the resources assuming two things. One we could get the resource and use it successfully. secondly there could be some error/exception while acquiring the resource. So the resource acquisition and usage should always happen inside a try block. The resource release should always be in the finally block. As this finally block will be executed always. Let us see a small code snippet to understand a little better.Using Try-finally to manage resources
//1. Let us first see how we should use a resource typically
TextReader tr = null;
try
{
//lets aquire the resources here
tr = new StreamReader(@"Files\test.txt");
//do some operations using resources
string s = tr.ReadToEnd();
Console.WriteLine(s);
}
catch (Exception ex)
{
//Handle the exception here
}
finally
{
//lets release the aquired resources here
if (tr != null)
{
tr.Dispose();
}
}
Putting 'using' in place to manage resources
The other way to do the same thing us to use
using
, this way we only have to think about the resource acquisition and the exception handling. the resource release will be done automatically because the resource acquisition is wrapped inside the using
block. Lets do the same thing we did above putting the using
block in place. //2. Let us now look at the other way we should do the same thing (Recommended version)
TextReader tr2 = null;
//lets aquire the resources here
try
{
using (tr2 = new StreamReader(@"Files\test.txt"))
{
//do some operations using resources
string s = tr2.ReadToEnd();
Console.WriteLine(s);
}
}
catch (Exception ex)
{
//Handle the exception here
}
Both the above approaches are essentially the same but the only difference is that in the first approach we have to release the resource explicitly where as in the second approach the resource release was done automatically.
using
block is the recommended way of doing such things as it will do the clean up even if the programmers forget to do it.
The use of '
using
' block is possible because the TextReader
class in the above example is implementingIDisposable
pattern. A note on Finalizers
Finalizers
are like destructors. they will get called whenever the object goes out of scope. We typically don't need to implement Finalizers
but if we are planning to implement IDisposable
pattern and at the same time we want the resource cleanup to happen when the local object goes out of scope then we will have to have a Finalizer
implemented in our class. class SampleClass
{
~SampleClass()
{
//This is a Finalizer
}
}
When and why we need to Implement IDisposable
Now we know how the resource acquisition and release should be done ideally. we also know that the recommended way of doing this is
using
statement. Now its time to see why we might need to know more about implementing the IDisposable
pattern ourselves.
Lets assume that we are writing a class which will be reused all across the project. This class will acquire some resources. To acquire these resources our class will be needing some managed objects(like in above example) and some unmanaged stuff too(like using a COM component or having some unsafe code with pointers).
Now since our class is acquiring resources, the responsibility of releasing these resources also lies with the class. Let us have the class with all the resource aquisition and release logic in place.
class MyResources
{
//The managed resource handle
TextReader tr = null;
public MyResources(string path)
{
//Lets emulate the managed resource aquisition
Console.WriteLine("Aquiring Managed Resources");
tr = new StreamReader(path);
//Lets emulate the unmabaged resource aquisition
Console.WriteLine("Aquiring Unmanaged Resources");
}
void ReleaseManagedResources()
{
Console.WriteLine("Releasing Managed Resources");
if (tr != null)
{
tr.Dispose();
}
}
void ReleaseUnmangedResources()
{
Console.WriteLine("Releasing Unmanaged Resources");
}
}
We have the class ready with the resource allocation and deallocation code. We also have the functions to use the class. Now we want to use this class following the guidelines earlier in this article. i.e.
- Using the object in a
try-finally
block, acquire resources in try block and release them in finally. - Using the object in a
using
block, the resource release will be done automatically. - The object when goes out of scope it should get disposed automatically as it was a local variable.
Implementing IDisposable
Now if we need to perform the clean up using while using our object and facilitate all the above mentioned functionalities we need to implement the
IDisposable
pattern. Implementing IDisposable
pattern will force us to have a Dispose
function.
Secondly if the user want to use the
try-finally
approach then also he can call this Dispose function and the object should release all the resources.
Lastly and most importantly, lets have a
Finalizer
that will release the unmanaged resources when the object goes out of scope. The important thing here is to do this finalize only if the programmer is not using the 'using
' block and not calling the Dispose
explicitly in a finally block.
For now lets create the stubs for these functions.
class MyResources : IDisposable
{
// ...
#region IDisposable Members
public void Dispose()
{
}
#endregion
~MyResources()
{
}
}
So to understand the possible scenario how this class might need disposal let us look at the following possible use cases for our class. we will also see what should be done in each possible scenario.
- The user will not do anything to relase the resource. We have to take care of releasing resource in finalizer.
- The user will use try-finally block. We need to do the clean up and ensure that finalizer will not do it again.
- The user will put a using 'block'. We need to do the clean up and ensure that finalizer will not do it again.
So here is the standard way of doing this Dispose business. It is also known as dispose pattern. Lets see how this should be done to achieve all we wanted.
public void Dispose()
{
// If this function is being called the user wants to release the
// resources. lets call the Dispose which will do this for us.
Dispose(true);
// Now since we have done the cleanup already there is nothing left
// for the Finalizer to do. So lets tell the GC not to call it later.
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (disposing == true)
{
//someone want the deterministic release of all resources
//Let us release all the managed resources
ReleaseManagedResources();
}
else
{
// Do nothing, no one asked a dispose, the object went out of
// scope and finalized is called so lets next round of GC
// release these resources
}
// Release the unmanaged resource in any case as they will not be
// released by GC
ReleaseUnmangedResources();
}
~MyResources()
{
// The object went out of scope and finalized is called
// Lets call dispose in to release unmanaged resources
// the managed resources will anyways be released when GC
// runs the next time.
Dispose(false);
}
So now the complete definition of our class looks like this. This time I have added some print messages to the methods so that we can see how things work when we use different approaches.
class MyResources : IDisposable
{
//The managed resource handle
TextReader tr = null;
public MyResources(string path)
{
//Lets emulate the managed resource aquisition
Console.WriteLine("Aquiring Managed Resources");
tr = new StreamReader(path);
//Lets emulate the unmabaged resource aquisition
Console.WriteLine("Aquiring Unmanaged Resources");
}
void ReleaseManagedResources()
{
Console.WriteLine("Releasing Managed Resources");
if (tr != null)
{
tr.Dispose();
}
}
void ReleaseUnmangedResources()
{
Console.WriteLine("Releasing Unmanaged Resources");
}
public void ShowData()
{
//Emulate class usage
if (tr != null)
{
Console.WriteLine(tr.ReadToEnd() + " /some unmanaged data ");
}
}
public void Dispose()
{
Console.WriteLine("Dispose called from outside");
// If this function is being called the user wants to release the
// resources. lets call the Dispose which will do this for us.
Dispose(true);
// Now since we have done the cleanup already there is nothing left
// for the Finalizer to do. So lets tell the GC not to call it later.
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
Console.WriteLine("Actual Dispose called with a " + disposing.ToString());
if (disposing == true)
{
//someone want the deterministic release of all resources
//Let us release all the managed resources
ReleaseManagedResources();
}
else
{
// Do nothing, no one asked a dispose, the object went out of
// scope and finalized is called so lets next round of GC
// release these resources
}
// Release the unmanaged resource in any case as they will not be
// released by GC
ReleaseUnmangedResources();
}
~MyResources()
{
Console.WriteLine("Finalizer called");
// The object went out of scope and finalized is called
// Lets call dispose in to release unmanaged resources
// the managed resources will anyways be released when GC
// runs the next time.
Dispose(false);
}
}
Let us use this class first with a
try-finally
block//3. Lets call out class using try-finally block
MyResources r = null;
try
{
r = new MyResources(@"Files\test.txt");
r.ShowData();
}
finally
{
r.Dispose();
}
The sequence of operations can be understood by these ouptut messages.
Aquiring Managed Resources
Aquiring Unmanaged Resources
This is a test data. / Some unmanaged data.
Dispose called from outside
Actual Dispose called with a True
Releasing Managed Resources
Releasing Unmanaged Resources
Let us use this class putting a
using
block in place//4. The using block in place
MyResources r2 = null;
using (r2 = new MyResources(@"Files\test.txt"))
{
r2.ShowData();
}
The sequence of operations can be understood by these ouptut messages.
Aquiring Managed Resources
Aquiring Unmanaged Resources
This is a test data. / Some unmanaged data.
Dispose called from outside
Actual Dispose called with a True
Releasing Managed Resources
Releasing Unmanaged Resources
Let us use this and leave the resource release to GC. the unmanaged resources should still be cleaned up when the finalizer gets called.
//5. Lets not do anything and the GC and take care of managed data
// we will let our finalizer to clean the unmanaged data
MyResources r3 = new MyResources(@"Files\test.txt");
r3.ShowData();
The sequence of operations can be understood by these ouptut messages.
Aquiring Managed Resources
Aquiring Unmanaged Resources
This is a test data. / Some unmanaged data.
Finalizer called
Actual Dispose called with a False
Releasing Unmanaged Resources
Points of Interest
Implementing
IDisposable
has always been a little confusing for the beginners. I have tried to jot down my understanding of when to implement this interface and what is the right way if implementing it. Although this article is written from a beginner's perspective, I hope this article has been informative and helpful to someone.
****************************************************************************************************************************
http://www.codeproject.com/Articles/269350/Destructors-vs-Finalizers-vs-Dispose-Pattern-Part?display=Print
I shall be talking about 3 topics by which we can clean up our mess created
in our programs provided in C# .NET world.
Luckily after spending long time reading all the articles and trying to figure out the answers, i thought about writing a blog post on the my learn points. I shall be explaining in detail about these topics and in next couple of posts as and when i dig more and more about it. I would not claim that the content in this article is extraordinary because i might have missed out an article which could be good. Its just that i could not find the information easily. Have tried my best to give as much in depth information as possible. So kindly leave a comment if i have left out any thing or wrongly interpreted.
Let me start off then
Most of the C# .NET developers do get confused about these 3 topics and i must say here that these topics are really important as you gain higher programming experience in C# .NET world.
Note: In this article, i shall use Dtor. for Destructors and Ctor. for Constructors.
So traditionally all these years, most of our smart developers in various native languages viz C, C++, pascal, etc. Used to write alot of code to ensure that the memory is cleaned up neatly though it may not be the perfect ones but atleast we can say some cleaning up was better than making it more mess or left undone. It was not easy to write efficient code to clean up our used resources. So you can think how memory management was most complex and worried topic.
To avoid heavy complexity in managing memory, .NET technology came as a boon to developers who used to spend day and nights for memory management earlier. In .NET, memory management in cleaning up is often taken care off by our beloved GC, yes we all know that, so nothing new. But we often fail to convince ourselves that just because GC is there, it is yet not perfect. Because after all its again a bunch of codes written with some AI (Artificial intelligence) put into it in taking care of memory efficiently.
Even though GC does most of the jobs in reclaiming the memory back consumed by our code, still there are times we need to make sure we explicitly write code to release memory or help GC in its job. Hence to do this, C# .NET provided us 3 ways. So lets dig deep into each of those ways:
“Don’t get surprised if you read or hear from some body saying that .NET does not support destructors.”
Lets get in detail about the Dtor., then:
Like C++, the signature for Dtor. is similar in C# as well. So as per that C# like C++, Dtor. has the same name as the class prefixed with ~ char. Unlike Ctor., Dtor. can’t have any Access Modifiers associated with it nor you can make an explicit call. This is because, this is the last method which shall be called automatically by the run time before the resource is getting actually cleaned up or marked for garbage collection and hence no body else should be allowed to call explicitly, hence in IL a family (protected) modifier is assigned to this method to make it available for subclass, so that run time can track dependencies down the object hierarchy.
Now lets look a bit deeper into Dtor. here, upon disassembling, below compiled code in IL the Dtor. has been changed to some thing else as seen in the below image:

As you can see from the above image, the IL shows that the Dtor. method has been converted to
Lets see whats inside this strange named method called

As you can see from the above image, the compiler has introduced try-finally block into the Dtor. method.
The compiler is making sure that no user/developer written code in the Dtor. throws exception or go unhandled, reason being that in no case the memory reclaiming process should face any exception getting raised i.e the GC expects that any thing on which it is reclaiming the memory should not throw any exceptions.
A point worth mentioning here that, even though upon any exceptions generated on an instance, which GC is reclaiming memory by finalizing it. GC shall ignore those exceptions which got generated but on the same time it stops reclaiming memory. So its very uncertain that when the left incomplete job is taken up again will be completed.
Now there is one strange method being called inside finally block if you look at the IL picture. This is because it explicitly notifying the GC to garbage collect this object. I shall talk about
Lets ask ourselves the following questions about Dtor here and see if we can answer it convincingly to ourselves first.
A point worth mentioning here is that, even if you wish to see
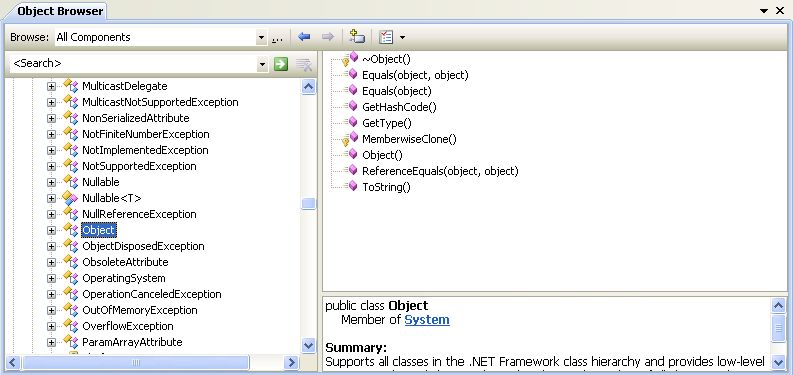
The same holds true for

So if you are really curious like me to see if there’s really an
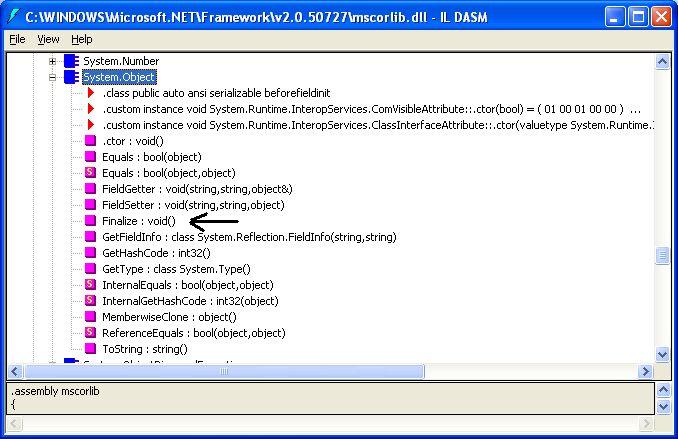
That’s all dear reader, i do hope you have got enough insight about Dtor. feature in C#.NET.
In the next issue, i might continue on Finalizers and finally on Dispose pattern in depth if i could find some thing interesting about it from my experiments.
Thanks
***********************************************************************************************
http://www.codeproject.com/Articles/2504/Understanding-Destructors-in-C
Bit History
I am sure you must be thinking now that “oh why re-inventing wheel?” or “Oh no not another same article which is available every where”. Honestly, these days i wanted to gain in depth knowledge on these topics and hence 3-4 days back i started reading many articles/blogs online in my free time. Upon reading so, i got many doubts which i started to ask myself. And i could not find answers for those self questions in one article/blog online. The information was so scattered, from Codeproject to Stackoverflow to CodeBetter to Codeguru and to couple of blogs.Luckily after spending long time reading all the articles and trying to figure out the answers, i thought about writing a blog post on the my learn points. I shall be explaining in detail about these topics and in next couple of posts as and when i dig more and more about it. I would not claim that the content in this article is extraordinary because i might have missed out an article which could be good. Its just that i could not find the information easily. Have tried my best to give as much in depth information as possible. So kindly leave a comment if i have left out any thing or wrongly interpreted.
Let me start off then
Most of the C# .NET developers do get confused about these 3 topics and i must say here that these topics are really important as you gain higher programming experience in C# .NET world.
Note: In this article, i shall use Dtor. for Destructors and Ctor. for Constructors.
Introduction
We know in programming world, when ever we write some thing or consume some thing in programs we are occupying some space which is a memory here. So its always been our headache for decades in programming world to clean up our mess, the reason i call it as mess is that no matter how carefully we write our codes, still there are many areas we might leave our footprints back which we fail to clean up.So traditionally all these years, most of our smart developers in various native languages viz C, C++, pascal, etc. Used to write alot of code to ensure that the memory is cleaned up neatly though it may not be the perfect ones but atleast we can say some cleaning up was better than making it more mess or left undone. It was not easy to write efficient code to clean up our used resources. So you can think how memory management was most complex and worried topic.
To avoid heavy complexity in managing memory, .NET technology came as a boon to developers who used to spend day and nights for memory management earlier. In .NET, memory management in cleaning up is often taken care off by our beloved GC, yes we all know that, so nothing new. But we often fail to convince ourselves that just because GC is there, it is yet not perfect. Because after all its again a bunch of codes written with some AI (Artificial intelligence) put into it in taking care of memory efficiently.
Even though GC does most of the jobs in reclaiming the memory back consumed by our code, still there are times we need to make sure we explicitly write code to release memory or help GC in its job. Hence to do this, C# .NET provided us 3 ways. So lets dig deep into each of those ways:
Destructors
This is not a feature which .NET supports but as a language C# has derived it from its predecessor C++ on which this language got evolved.“Don’t get surprised if you read or hear from some body saying that .NET does not support destructors.”
Lets get in detail about the Dtor., then:
Like C++, the signature for Dtor. is similar in C# as well. So as per that C# like C++, Dtor. has the same name as the class prefixed with ~ char. Unlike Ctor., Dtor. can’t have any Access Modifiers associated with it nor you can make an explicit call. This is because, this is the last method which shall be called automatically by the run time before the resource is getting actually cleaned up or marked for garbage collection and hence no body else should be allowed to call explicitly, hence in IL a family (protected) modifier is assigned to this method to make it available for subclass, so that run time can track dependencies down the object hierarchy.
Now lets look a bit deeper into Dtor. here, upon disassembling, below compiled code in IL the Dtor. has been changed to some thing else as seen in the below image:
class MyClass
{
~MyClass() { }
}
As you can see from the above image, the IL shows that the Dtor. method has been converted to
Finalize()
method, well this is how the Run time
identifies and calls it before really the object is garbage collected. Hence i
said before that Dtor. is not a feature .NET is offering to us but rather C# is.
This way this object can be marked for finalization. Don’t get worried about
Finalization now, i shall explain later.Lets see whats inside this strange named method called
Finalize()
AKA the Dtor.As you can see from the above image, the compiler has introduced try-finally block into the Dtor. method.
The compiler is making sure that no user/developer written code in the Dtor. throws exception or go unhandled, reason being that in no case the memory reclaiming process should face any exception getting raised i.e the GC expects that any thing on which it is reclaiming the memory should not throw any exceptions.
A point worth mentioning here that, even though upon any exceptions generated on an instance, which GC is reclaiming memory by finalizing it. GC shall ignore those exceptions which got generated but on the same time it stops reclaiming memory. So its very uncertain that when the left incomplete job is taken up again will be completed.
Now there is one strange method being called inside finally block if you look at the IL picture. This is because it explicitly notifying the GC to garbage collect this object. I shall talk about
Finalize()
method
later.Lets ask ourselves the following questions about Dtor here and see if we can answer it convincingly to ourselves first.
- Why is that i see a Try-Catch/Finally block in the transformed Dtor method?
- Can i fool the compiler by providing a custom Try-Catch/Finally block in the code?
- I see
Object.Finalize()
method being called in Finally. Why?
Object.Finalize()
notifes the GC to garbage collect this object.
But dont get relaxed reading this, doing finalization is not as easy as you
think by GC. Hence in .NET GC is non-deterministic in nature. Hence it has to be
called at the end of your object life cycle.- Why can not i call directly this
Finalize()
method than using Dtor.?
Finalize()
method is a protected method of Object class and C#
does not allow you to call that method. Try it and you get a compiler error. So
its all the trick Compiler is playing. The reason is that calling
Finalize()
is not recommended because it deals with alot complex
operations internally. If allowed to call it explicitly in c#, a developer can
call it when ever and at any time he/she wants. This will overload the whole
system rather than improving it. Hence to avoid it, C# does ask you to use
Dtor.- Since i can’t call
Finalize()
, it should have been private but why it is protected?
System.Object
right? So, if Finalize()
is private,
then Finalization of that object is not possible and every child object has to
provide its own implementation of Finalize if this was true. This adds alot of
overhead. To reduce all this craziness, its made protected and C# does not allow
you to use it explicitly.A point worth mentioning here is that, even if you wish to see
Object.Finalize()
method via Visual studios Object Browser, then
you can’t. You can only see ~Object()
method which is kind of an
alias for Object.Finalize()
as you can see from below image:The same holds true for
System.object
source code in visual
studio too. Although the Objects Dtor. is also missing here as you can see from
below image :So if you are really curious like me to see if there’s really an
Finalize()
method in System.Object
class, then
disassembling is the only way as shown below:That’s all dear reader, i do hope you have got enough insight about Dtor. feature in C#.NET.
In the next issue, i might continue on Finalizers and finally on Dispose pattern in depth if i could find some thing interesting about it from my experiments.
Thanks
***********************************************************************************************
http://www.codeproject.com/Articles/2504/Understanding-Destructors-in-C
http://www.codeproject.com/Articles/319826/IDisposable-Finalizer-and-SuppressFinalize-in-Csha
No comments:
Post a Comment